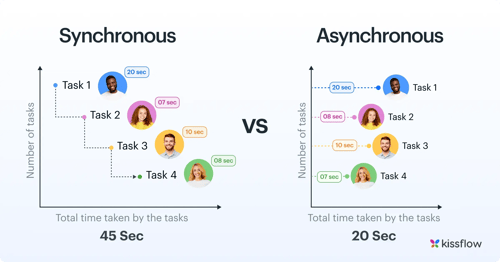
- >
- Application Development >
- Synchronous vs. Asynchronous Programming: Complete Guide of 2025
Synchronous vs. Asynchronous Programming: Complete Guide of 2025
Team Kissflow
Updated on 28 Apr 2025 • 16 min read
Introduction to Synchronous and Asynchronous Programming
The debate between synchronous vs. asynchronous programming has significantly influenced modern software development. From microservices architecture and API design to scalable enterprise solutions, understanding these two paradigms is essential for building efficient and reliable systems.
This guide provides a detailed overview, covering fundamentals, key differences, real-world applications, tools, and emerging trends in 2025.
Choosing between synchronous and asynchronous programming can directly impact performance, scalability, and operational efficiency. For IT Managers, Directors of IT, VP Apps, and IT Heads, understanding these approaches is key to making strategic decisions aligned with business objectives.
We’ll explore:
- Core differences between synchronous and asynchronous programming
- Specific use cases and challenges of each approach
- Best practices for effective implementation
We’ll also highlight how Kissflow’s low-code platform applies asynchronous programming to improve workflow efficiency, accelerate deployments, and enhance scalability in enterprise environments.
This guide is a reference point for making informed programming choices supporting long-term business goals.
What is Synchronous and Asynchronous Programming: Key Differences Explained
What is Synchronous Programming? Key Concepts, JavaScript Example, and Use Cases
Synchronous programming follows a linear and sequential execution model, where each task must be completed before the next one begins. This approach ensures clarity, predictable task flow, and easier debugging, but it can be inefficient for tasks requiring long waiting periods or heavy I/O operations.
Key Characteristics of Synchronous Programming
- Blocking Execution: Tasks block the main thread until completion.
- Linear Flow: Operations occur in a predictable, step-by-step order.
- Simplified Debugging: Errors are easier to trace and address.
How Synchronous Programming Works
- The first task starts and is completed before the next begins.
- Subsequent tasks execute in order, without overlap.
Advantages of Synchronous Programming
- Clear and predictable task flow.
- Easier debugging and tracing errors.
- Effective for small-scale operations requiring step-by-step execution.
Disadvantages of Synchronous Programming
- Limited scalability with increasing task volume.
- Inefficient resource utilization for I/O-heavy tasks.
- Bottlenecks in real-time operations.
Real-World Examples of Synchronous Programming
- Financial Transaction Systems: Tasks execute sequentially to ensure data integrity.
- Batch Data Processing: Linear workflows simplify large-scale data operations.
What is Asynchronous Programming?
Asynchronous programming allows multiple tasks to execute independently and concurrently, ensuring that time-consuming operations such as API calls, file uploads, or network requests do not block the execution of other tasks. This approach is essential for high-performance applications development requiring scalability and responsiveness.
Key Characteristics of Asynchronous Programming
- Non-Blocking Execution: Tasks execute without waiting for previous tasks to finish.
- Concurrent Operations: Multiple tasks can run simultaneously, improving efficiency.
- Event-Driven Architecture: Event loops and callbacks handle task completion and continuation.
How Asynchronous Programming Works?
- Task 1 begins execution.
- Task 2 starts without waiting for Task 1 to complete.
- Both tasks finish independently, optimizing resource usage.
Advantages of Asynchronous Programming
- Efficient Resource Utilization: Avoids idle waiting by maximizing system resources.
- Supports Thousands of Concurrent Tasks: Handles large volumes of operations without slowing down.
- Faster I/O-Bound Operations: Optimized for tasks involving file systems, databases, or network requests.
Disadvantages of Asynchronous Programming
- Complex Debugging: Non-linear execution makes tracing errors challenging.
- Callback Hell: Poorly structured code can become unmanageable with nested callbacks.
- Error Management Challenges: Handling asynchronous errors requires careful implementation.
Real-World Examples of Asynchronous Programming
- Live Chat Applications: Real-time communication systems rely on asynchronous operations to maintain responsiveness.
- Real-Time Data Analytics: Platforms process and display live data streams efficiently using asynchronous workflows.
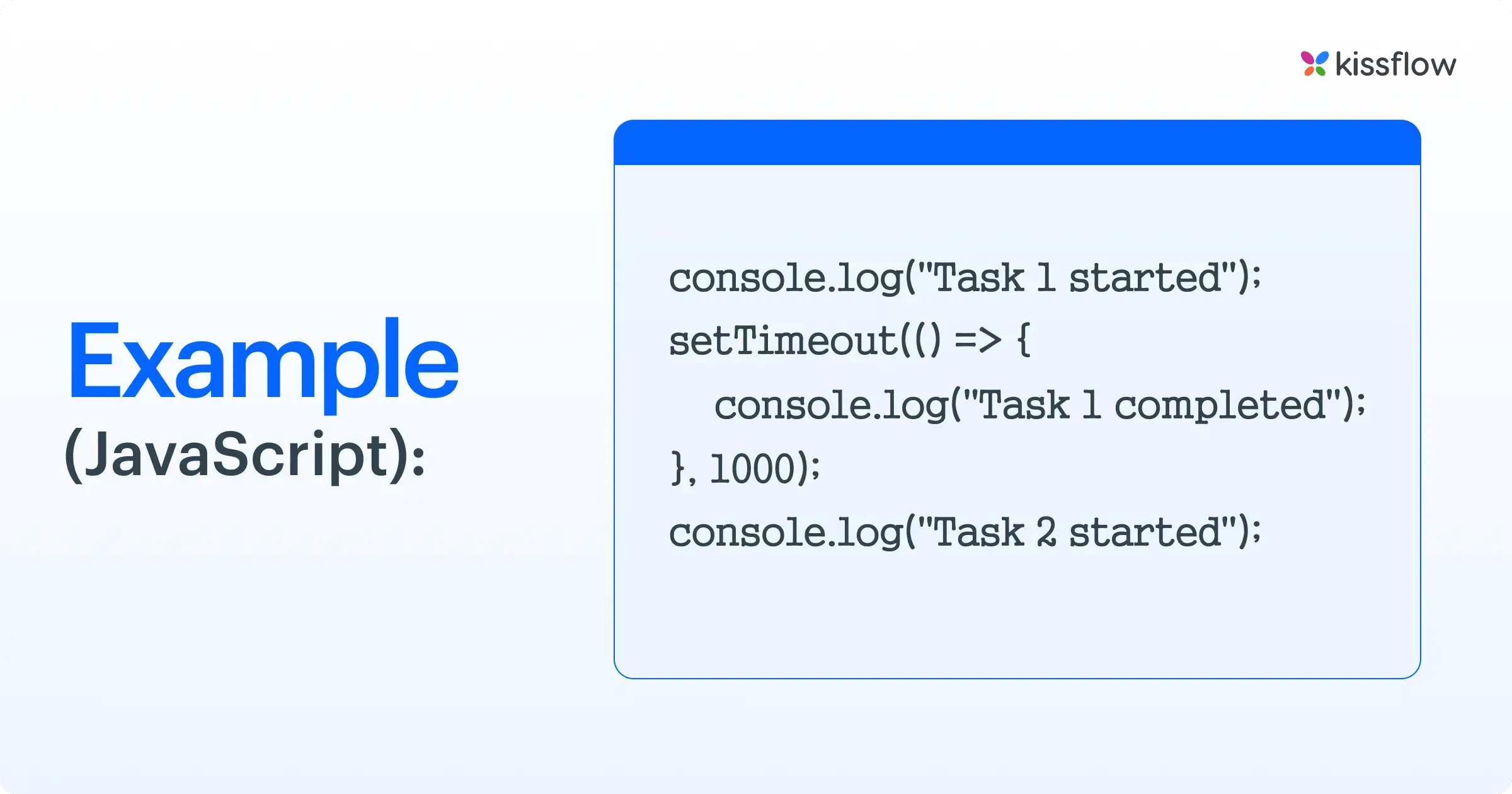
Core Differences Between Synchronous and Asynchronous Programming
Criteria | Synchronous Programming | Asynchronous Programming |
---|---|---|
Execution Model | Tasks executed one by one | Tasks run independently |
Responsiveness | Blocking | Non-blocking |
Resource Utilization | Higher resource usage | Optimized resource allocation |
Scalability | Limited | Highly scalable |
Use Cases | Simple, sequential tasks | Complex, parallel tasks |
Performance | Slower with I/O | Faster with I/O |
Best Fit | Predictable Tasks | Real-Time Systems |
Key Takeaway: Synchronous programming is best for straightforward, sequential tasks, while asynchronous programming excels in scenarios demanding responsiveness and concurrency.
The Benefits of Synchronous Programming: Advantages for Developers
Synchronous programming remains a reliable approach for many software development tasks, offering clarity, predictability, and efficiency. While it may not always be suitable for large-scale concurrent systems, it excels in scenarios requiring linear execution flows and simplified debugging.
Advantages of Synchronous Programming
Predictable performance
Tasks execute sequentially, ensuring that each operation completes fully before the next one begins. This structured approach minimizes unexpected behaviors caused by overlapping operations, leading to consistent resource utilization and smoother execution flows. The clear task flow also prevents bottlenecks and delays, ensuring that resources such as CPU cycles and memory are effectively utilized.
Simplicity of the codebase.
Synchronous programming allows developers to write code in a linear and intuitive fashion, reducing the reliance on callbacks or promises. This straightforward structure not only reduces the complexity of the code but also makes it easier to manage and maintain. Developers can focus on core application development platform logic without being distracted by intricate concurrency controls, resulting in cleaner and more manageable code.
Debugging
With tasks executed in a strict sequential order, developers can logically trace the execution flow and pinpoint errors with greater accuracy. This reduces the time spent identifying and resolving bugs, leading to faster issue resolution and improved productivity across the development cycle.
Code readability
Its linear task execution promotes a clear and logical flow, making the codebase easier to understand. Developers can quickly grasp the intent and logic of the application without navigating through complex asynchronous workflows. This clarity not only benefits individual developers but also enhances team collaboration, as multiple contributors can work on the same codebase with minimal friction.
Task synchronization
By enforcing a predictable order of task execution, it effectively avoids common pitfalls such as race conditions, deadlocks, or inconsistencies in task outcomes. Applications built with this approach are inherently more stable and reliable, particularly in environments where task order and execution consistency are critical.
In real-world scenarios, synchronous programming is well-suited for tasks that require strict sequential execution, such as financial transaction systems, batch data processing, or configuration scripts. These systems rely on the predictability and reliability of synchronous execution to ensure data integrity and operational accuracy. For these use cases, synchronous programming stands out as a practical and dependable choice.
The Benefits of Asynchronous Programming: Why It’s Essential for Modern Software Development
Asynchronous programming has become an essential paradigm in modern software development, enabling applications to handle multiple tasks simultaneously without blocking the main execution thread. This approach is particularly valuable in environments where I/O operations, network requests, or database interactions play a significant role. Its growing adoption can be attributed to the substantial advantages it offers in terms of performance, scalability, and user responsiveness.
Advantages of Synchronous Programming
Faster performance
By allowing tasks to run concurrently rather than waiting for each to complete sequentially, the overall execution time is significantly reduced. This advantage becomes especially clear in applications involving frequent I/O operations, such as fetching data from APIs, reading from databases, or processing file uploads. These tasks can proceed in parallel without delaying other critical operations, resulting in improved efficiency.
Scalability
Modern applications, particularly web platforms, must accommodate thousands of concurrent requests without degrading performance. By enabling tasks to run independently, asynchronous workflows allow systems to scale efficiently and remain responsive, even under heavy loads. This scalability is essential for applications like online marketplaces, cloud services, or live-streaming platforms, where uninterrupted service is critical.
Enhanced responsiveness
In asynchronous systems, tasks that require extended processing times can run in the background without affecting the application’s primary functions. This ensures that user interfaces remain smooth and interactive, even during resource-intensive operations. Applications that rely on real-time interactions, such as chat platforms, live dashboards, or gaming servers, benefit immensely from this approach.
Error handling
While traditional synchronous programming can allow errors to propagate across tasks and disrupt the entire application, asynchronous systems isolate errors within individual tasks. This isolation simplifies debugging and ensures that failures in one task do not compromise the stability of the entire application.
Resource efficiency
Instead of keeping threads idle while waiting for tasks to complete, asynchronous workflows allow resources to be reassigned to other operations. This dynamic allocation minimizes idle time and ensures optimal hardware utilization, which is particularly important in cloud computing and serverless architectures.
In practical terms, asynchronous programming is widely used in scenarios requiring real-time communication, high-concurrency systems, and large-scale data processing. Applications like live chat platforms, analytics dashboards, and backend services for mobile apps rely on asynchronous workflows to deliver smooth and reliable performance.
Challenges of Synchronous and Asynchronous Programming
In software development, choosing between synchronous and asynchronous programming requires a deep understanding of their respective challenges. Both approaches offer unique advantages, but each also comes with inherent limitations that can impact performance, scalability, and error management. Recognizing these challenges is essential for making informed architectural and development decisions.
Challenges of Synchronous Programming
Sequential execution model
Synchronous programming follows a sequential execution model, where tasks are processed one after another. While this approach simplifies the development process and improves code readability, it introduces significant limitations in complex or high-concurrency environments.
Call blocking
One major challenge is the presence of blocking calls. In synchronous programming, each task must fully complete before the next one begins, which can lead to idle resources and poor system performance when tasks involve long waiting periods, such as database queries or network requests.
Scalability
Another limitation is scalability. Scaling synchronous systems often requires significant resources, and adding new processes or threads without disrupting existing ones becomes increasingly difficult as workloads grow. This makes synchronous programming less suitable for systems requiring high concurrency.
Error handling
Error handling in synchronous programming can also become problematic, especially when multiple dependent tasks are involved. An error in one task can halt the execution flow entirely, requiring developers to carefully manage dependencies and error propagation across tasks.
Challenges of Asynchronous Programming
Asynchronous programming, on the other hand, allows tasks to execute concurrently, improving responsiveness and resource utilization. However, this flexibility comes with its own set of challenges, primarily around complexity. Writing and managing asynchronous code requires developers to think differently, often involving advanced concepts like event loops, callbacks, or promises. This can create a steeper learning curve, especially for teams more familiar with synchronous models.
Debugging asynchronous code
This poses another significant challenge. Since tasks do not execute in a predictable sequence, tracing the flow of execution to identify errors can be complex. Developers may find it harder to pinpoint where failures occur, particularly in systems with heavy concurrency and interdependent tasks.
Error handling
Error handling is equally challenging in asynchronous workflows. Errors can be difficult to isolate when multiple tasks are running concurrently, and failures in one task might propagate unpredictably across others. Properly managing errors in asynchronous systems often requires additional frameworks or design patterns to maintain stability.
Balancing the Challenges
Both synchronous and asynchronous programming have their place in software development, and understanding their challenges is essential for choosing the right approach. Synchronous programming excels in scenarios requiring simplicity, predictability, and strict task order, while asynchronous programming thrives in systems requiring high concurrency, responsiveness, and non-blocking operations.
By acknowledging these limitations and implementing best practices, developers can mitigate the risks associated with both paradigms, leading to more reliable and efficient applications.
When to use synchronous vs. asynchronous programming
Choosing between synchronous and asynchronous programming depends on the specific needs of your application, including its structure, performance requirements, and scalability goals. Each approach serves distinct use cases, and understanding their ideal scenarios helps in making informed architectural decisions.
When to use synchronous programming
Synchronous programming is ideal for applications requiring a simple, predictable execution flow where tasks must be processed sequentially. This approach works well for scenarios where each task relies on the successful completion of the previous one.
For instance, synchronous programming is well-suited for single-threaded applications, batch processing systems, or scripts that perform configuration tasks. These environments benefit from the clarity and simplicity offered by synchronous task execution, as each operation is completed before the next begins. It is also a strong choice for small-scale applications where the overhead of managing concurrency isn’t justified.
However, synchronous programming may not scale effectively in systems requiring high concurrency or where long wait times (e.g., I/O operations) are common.
When to use asynchronous programming
Asynchronous programming shines in scenarios requiring high concurrency, parallel processing, or the ability to handle multiple independent tasks simultaneously. Applications that depend on frequent I/O operations, such as database queries, API calls, or file uploads, benefit significantly from this approach.
For example, web servers that must handle thousands of simultaneous client requests, real-time communication platforms like live chat applications, or streaming services rely heavily on asynchronous workflows. By ensuring non-blocking execution, asynchronous programming prevents bottlenecks and keeps applications responsive under heavy loads.
This approach is particularly effective for scalable systems, where resource utilization and efficient task management are critical to maintaining performance.
In practice, many modern applications combine both approaches, using synchronous programming for task-critical workflows and asynchronous programming for I/O-heavy operations. The choice ultimately depends on the application’s architecture and specific performance requirements.
Tools and Frameworks for Synchronous and Asynchronous Programming
The choice of tools and frameworks plays a crucial role in implementing synchronous and asynchronous programming paradigms effectively. Different programming languages and ecosystems offer robust support for both approaches, enabling developers to build efficient and scalable applications.
Tools and frameworks for synchronous programming
Synchronous programming thrives in scenarios requiring linear task execution and predictable workflows. Several languages and frameworks excel in supporting synchronous workflows:
Java offers a rich ecosystem of libraries and frameworks tailored for synchronous programming. Its thread-based model and built-in concurrency utilities make it a reliable choice for systems requiring sequential task execution and resource management.
C# provides strong support for synchronous programming with constructs like Thread.Sleep and lock mechanisms to manage sequential operations effectively. Its structured approach simplifies debugging and ensures clear execution flow.
Node.js, traditionally known for asynchronous programming, also supports synchronous workflows. It enables developers to build event-driven systems with controlled task execution, particularly in scenarios requiring simpler, sequential logic.
While synchronous programming simplifies debugging and task flow management, it may not scale well under heavy concurrency or resource-intensive tasks.
Tools and Frameworks for Asynchronous Programming
Asynchronous programming excels in environments requiring high concurrency, non-blocking I/O operations, and parallel task execution. The following tools and frameworks provide strong support for asynchronous workflows:
Java offers powerful concurrency constructs such as Futures and CompletableFutures, which enable developers to handle non-blocking operations efficiently. These constructs simplify asynchronous task management, ensuring scalable performance.
C# has built-in support for asynchronous workflows through the async/await keywords, which simplify writing and managing non-blocking code. This feature ensures smooth task execution without freezing the main application thread.
Node.js, widely regarded as a leader in asynchronous programming, is built around an event-driven, non-blocking architecture. Its libraries and frameworks, such as Express.js, make it an ideal choice for building real-time web applications, APIs, and high-traffic services.
Asynchronous tools and frameworks empower developers to build applications that handle thousands of concurrent tasks efficiently while maintaining optimal responsiveness and resource utilization.
Choosing the Right Tool for the Task
The selection between synchronous and asynchronous tools depends on the specific requirements of the application. For tasks requiring strict sequential execution, synchronous tools like Java and C# provide clarity and simplicity. On the other hand, applications demanding high scalability and responsiveness benefit from asynchronous frameworks like Node.js and advanced concurrency libraries in Java and C#.
Synchronous vs. Asynchronous Programming in Web Development
In web development, both synchronous and asynchronous programming serve distinct purposes, each addressing specific application requirements. The choice between them depends on factors such as application complexity, traffic volume, and performance needs.
Synchronous programming is best suited for simple web applications where tasks execute in a sequential order and user interactions are minimal. It works effectively in scenarios where operations must be completed one step at a time, such as form submissions, configuration tasks, or workflows requiring strict execution order. This approach ensures clarity in task flow but may lead to delays in systems handling heavy loads or resource-intensive operations.
On the other hand, asynchronous programming is essential for high-traffic, complex web applications that demand scalability and real-time responsiveness. Tasks like API requests, database queries, or file uploads benefit significantly from asynchronous workflows, as they allow multiple operations to run concurrently without blocking the main execution thread. This ensures that user interfaces remain responsive and system resources are efficiently utilized, even under heavy demand.
In practice, modern web development often combines synchronous and asynchronous paradigms. Critical workflows requiring strict execution order leverage synchronous programming, while asynchronous workflows handle tasks involving concurrency, network communication, and data-intensive processes.
Case Studies: Real-World Applications of Synchronous and Asynchronous Programming
Understanding how industry leaders leverage synchronous and asynchronous programming paradigms provides valuable insights into their practical applications, benefits, and challenges. Below are two prominent examples demonstrating how these approaches coexist in real-world systems.
Uber: Asynchronous for Real-Time Responsiveness, Synchronous for Critical Processes
Uber's ride-sharing platform heavily depends on asynchronous programming to handle the high concurrency needed for real-time matching between drivers and riders. Using Node.js and an event-driven architecture, Uber efficiently manages thousands of concurrent ride requests, real-time GPS tracking, and fare calculations without blocking primary operations.
However, critical workflows such as payment processing and ride completion logs often rely on synchronous programming to ensure sequential, error-free execution. These tasks require data consistency and must occur in a controlled, step-by-step manner to avoid discrepancies.
In this hybrid approach, Uber balances asynchronous operations for scalability and responsiveness with synchronous processes for data integrity and reliability.
Netflix: Combining Asynchronous APIs with Synchronous Processing
Netflix’s streaming service combines asynchronous programming for scalability and responsiveness with synchronous workflows for critical backend processes.
The platform utilizes asynchronous APIs in its content delivery pipeline to handle millions of concurrent connections without bottlenecks. Technologies like Netty and non-blocking I/O models allow Netflix to fetch and deliver video content across global regions easily.
However, specific backend tasks such as payment verification, content metadata updates, and billing operations follow a synchronous execution model. These tasks prioritize data accuracy and require sequential processing to ensure consistency across distributed systems.
This balanced combination enables Netflix to deliver smooth streaming experiences while maintaining reliability in its core business processes.
Best Practices for Synchronous Programming
Synchronous programming works best when tasks must be executed sequentially, with a clear order of operations. To maximize its efficiency:
Developers should minimize blocking calls, limiting them to scenarios where waiting for a resource or task is unavoidable. Excessive blocking can result in resource underutilization and reduced system responsiveness. Tasks in synchronous workflows should remain simple and focused, avoiding overly complex logic or dependencies that can make debugging and maintenance challenging. Additionally, thorough testing and validation are essential to ensure sequential workflows execute correctly without introducing cascading failures. Unit and integration tests are critical in identifying bottlenecks or unintended behaviors early in the development cycle.
Synchronous programming excels in applications requiring strict execution orders, such as batch data processing or transaction validation workflows.
Best Practices for Asynchronous Programming
Asynchronous programming thrives in systems requiring high concurrency and non-blocking operations. Effective implementation starts with non-blocking I/O operations wherever possible. This approach allows tasks to continue executing while waiting to complete network calls, database queries, or file operations, optimizing resource utilization.
To manage task execution order and ensure predictability, developers should rely on mechanisms such as Promises (JavaScript) or Futures (Java and C#). These constructs help structure asynchronous tasks cleanly, reducing the risks of callback chains or "callback hell."
Comprehensive testing strategies are equally crucial in asynchronous workflows. Since tasks often execute concurrently, ensuring reliable error handling and managing task dependencies through unit and integration testing is critical.
Lastly, performance benchmarking should be a regular practice in asynchronous workflows. While asynchronous systems generally perform better under high load, developers must measure performance across key metrics such as response time, concurrency limits, and resource allocation to address any bottlenecks proactively.
Emerging trends in Synchronous and Asynchronous Programming (2025 and Beyond)
As technology advances, synchronous and asynchronous programming paradigms continue adapting to meet the demands of modern software systems. These trends reflect a shift towards more efficient architectures, scalable workflows, and cutting-edge computational models that redefine task execution.
One prominent trend is the rise of serverless architectures, which have gained significant traction for synchronous and asynchronous programming workflows. Serverless computing abstracts infrastructure management, allowing developers to focus purely on code execution. In synchronous workflows, serverless architectures optimize resource allocation for sequential tasks, ensuring predictable execution and minimal latency. In asynchronous workflows, serverless platforms enable event-driven designs where tasks trigger independently, enhancing scalability and concurrency without the constraints of fixed infrastructure.
Another transformative trend is the increasing potential of quantum computing. By leveraging the principles of quantum mechanics, these systems can handle complex calculations and massive data sets far beyond the capabilities of classical computers. For synchronous programming, quantum algorithms may enable linear execution workflows to process exponentially larger datasets more efficiently. In asynchronous programming, quantum systems could manage unprecedented levels of concurrency, enabling tasks to run parallel across quantum states. While still in its early stages, quantum computing is poised to redefine performance benchmarks for both paradigms.
Looking ahead, the integration of artificial intelligence (AI) and machine learning (ML) into synchronous and asynchronous workflows will further enhance their capabilities. AI-driven systems will optimize task scheduling, predict resource bottlenecks, and improve error detection, making both paradigms smarter and more adaptive.
In addition, the focus on energy-efficient programming is set to grow. With sustainability becoming a priority, synchronous and asynchronous systems will increasingly emphasize optimized resource utilization to reduce energy consumption without compromising performance.
Asynchronous programming will also see deeper integration into microservices architectures, enabling distributed systems to process tasks independently across services. Meanwhile, synchronous workflows will remain essential for transactional systems requiring precision and order.
These trends indicate a future where synchronous and asynchronous programming coexist, each playing to its strengths while leveraging advancements in serverless computing, quantum processing, and intelligent automation to create highly efficient, scalable, and future-ready systems.
How AI and ML are transforming synchronous and asynchronous programming
Artificial Intelligence (AI) and Machine Learning (ML) are revolutionizing synchronous and asynchronous programming by introducing smarter task scheduling, resource allocation, and error management.
In synchronous programming, AI optimizes execution order and resource utilization, ensuring efficient task flow and reducing idle time. It helps predict dependencies and manage resources for tasks requiring strict sequential execution, such as transactional systems.
AI enhances concurrency management, task prioritization, and anomaly detection in asynchronous programming. ML algorithms predict workload spikes, optimize queue handling, and automate error recovery, making systems more resilient and scalable.
Both paradigms benefit from AI-driven predictive analytics, offering insights into performance bottlenecks and optimization opportunities. As these technologies advance, they will continue to drive efficiency, scalability, and intelligence in software development.
Why choose Kissflow for synchronous and asynchronous processes?
Kissflow is a low-code platform designed to simplify the development of synchronous and asynchronous workflows. Its intuitive drag-and-drop interface empowers users, regardless of technical expertise, to build and manage sophisticated workflows efficiently without extensive programming knowledge.
Simplifying sync and async processes with Kissflow low-code
Kissflow streamlines the complexities of managing synchronous and asynchronous workflows through its user-friendly design and powerful integrations. Users can easily create and oversee workflows, ensuring tasks are executed reliably and efficiently.
- Effortless Workflow Creation: The platform’s visual interface allows users to design workflows quickly, reducing dependency on IT teams.
- EEfficient Task Management: Synchronous and asynchronous tasks are managed seamlessly, enhancing productivity and clarity.
- ESmooth Integrations: Kissflow connects with popular CRM, ERP, and other enterprise systems, enabling smooth data flow and process automation across platforms.
How Kissflow leverages asynchronous programming
Kissflow’s low-code platform is engineered to handle the complexities of asynchronous programming, delivering optimal performance even in high-demand scenarios.
- Scalability: Easily manage thousands of tasks concurrently without risking performance slowdowns.
- Responsiveness: Long-running operations no longer block critical workflows, keeping processes agile and efficient.
- Faster Deployments: Streamlined task execution ensures minimal delays and accelerated go-to-market timelines.
Why Kissflow stands out
Kissflow combines simplicity, scalability, and integration capabilities to make synchronous and asynchronous workflow management accessible to everyone. Its low-code approach eliminates technical barriers, empowering teams to build, optimize, and deploy workflows quickly.
Frequently Asked Questions (FAQs)
What is Synchronous?
Synchronous refers to processes or actions that occur simultaneously or in real-time, requiring each step to complete before the next one begins. In technology and communication, it often describes tasks or operations where responses are immediate and executed in a sequential, predictable order. For example, a video call is synchronous because both parties interact and respond in real-time.
What is Asynchronous?
Asynchronous refers to processes or actions that occur independently of a fixed schedule, allowing tasks to proceed without waiting for others to complete. This enables flexibility, as responses or actions can happen at different times. In technology, asynchronous operations allow systems to perform multiple tasks simultaneously without blocking. For example, sending an email is asynchronous since the recipient can read and respond at their convenience.
3. What is the key difference between synchronous and asynchronous programming?
Synchronous programming executes tasks sequentially, meaning each task must complete before the next one starts. It's ideal for tasks requiring strict order and immediate feedback. In contrast, asynchronous programming allows multiple tasks to run independently without waiting for each other to complete, improving efficiency and responsiveness, especially in I/O-heavy operations or real-time data handling.
4. When should I choose asynchronous programming over synchronous programming?
Asynchronous programming is best suited for tasks involving network requests, database queries, or file operations where waiting time is significant. It shines in applications requiring scalability, like chat apps, live dashboards, or data streaming services. Conversely, synchronous programming works better for simple scripts, single-threaded tasks, or scenarios requiring strict execution order.
5. How does Kissflow's low-code platform support asynchronous workflows?
Kissflow's low-code platform enables organizations to build asynchronous workflows with minimal coding effort. It offers pre-built templates, drag-and-drop functionalities, and seamless integrations with third-party tools. This empowers teams to handle complex workflows, automate repetitive tasks, and ensure data consistency across systems efficiently.
6. Can synchronous and asynchronous programming coexist in the same application?
Yes, they can coexist. Many modern applications use a hybrid approach where synchronous programming manages straightforward, sequential tasks, while asynchronous programming handles non-blocking tasks like network requests or background processing. This approach balances simplicity and scalability.
7. What are the key benefits of adopting asynchronous workflows in enterprise applications?
Asynchronous workflows enhance application responsiveness, reduce processing bottlenecks, and allow tasks to run in parallel. They are particularly beneficial for managing large-scale data processing, improving system scalability, and enabling real-time updates without degrading system performance. With platforms like Kissflow, businesses can seamlessly integrate these workflows into their operations.
8. How does synchronous communication differ from asynchronous communication in APIs?
In synchronous APIs, the client sends a request and waits for an immediate response from the server before proceeding with the next task. This approach is ideal for operations requiring real-time confirmation, such as payment transactions or authentication requests.
On the other hand, asynchronous APIs allow the client to send a request and continue executing other tasks without waiting for an immediate response. Once the server processes the request, it notifies the client (via callbacks, webhooks, or polling). Asynchronous APIs are more efficient for long-running tasks, like data processing or file uploads, as they prevent blocking resources.
9. Which is better: synchronous or asynchronous for enterprise applications?
The choice between synchronous and asynchronous approaches depends on the application's requirements:
Synchronous: Best for real-time operations requiring immediate feedback, such as login verification or financial transactions.
Asynchronous: Ideal for long-running tasks, heavy data processing, and scenarios where scalability and responsiveness are crucial, such as report generation, background jobs, or cloud-based microservices. In most enterprise applications, a hybrid approach combining synchronous and asynchronous workflows is the optimal choice. This ensures critical operations remain reliable and time-sensitive, while background tasks are efficiently handled without performance bottlenecks.
Modern platforms like Kissflow offer built-in support for both paradigms, enabling enterprises to select the right approach based on their specific needs.
In most enterprise applications, a hybrid approach combining synchronous and asynchronous workflows is the optimal choice. This ensures critical operations remain reliable and time-sensitive, while background tasks are efficiently handled without performance bottlenecks.
Modern platforms like Kissflow offer built-in support for both paradigms, enabling enterprises to select the right approach based on their specific needs.
Bringing It All Together: Synchronous vs. Asynchronous
Choosing between synchronous and asynchronous programming depends on your application's goals, resource requirements, and scalability needs. For IT leaders, understanding these paradigms is critical for building reliable and efficient applications.
With Kissflow's low-code platform, enterprises can fully leverage asynchronous programming to streamline workflows, reduce bottlenecks, and accelerate innovation.
Explore how Kissflow can empower your team to build scalable, efficient, and future-ready applications today!
Related Articles
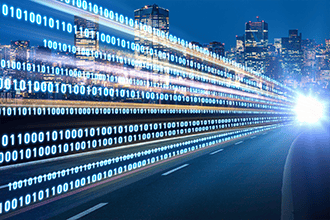
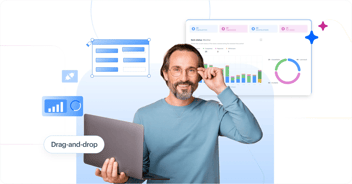